Parameters
All parameters explained at one place.
A string is a sequence of characters (letters, numbers, symbols, and spaces) used to represent text.
A float, or floating-point number, is a type of number that allows for fractional values. Floats are used to represent real numbers and support a wider range of values by including a decimal point. They are especially useful for scientific calculations and measurements where precision is essential.
A boolean is a data type that can hold one of two possible values: true
or false
. Booleans are used to represent binary conditions, such as yes/no, on/off, or true/false. They are fundamental in control structures like conditionals and loops.
A Lua table is like a container that can hold different kinds of things together. Think of it as a box where you can store items. The items can be numbers, words, other tables, or anything else. In our case, it's parameters.
If you see a question mark next to a parameter, it means it's optional, or you have a choice.
Name of heist
Define the name of the heist.
CFG.Heists["Pacific Bank"] = {
-- params
}
RequiredCops
Number of cops required to interact with the heist.
RequiredCops = 4
AllowPolice
This parameter is optional.
Allows defined jobs to interact with stuff inside the heist.
AllowPolice = true
Cooldown
Cooldown period in minutes, starts counting when START function is called.
Cooldown = 60
CooldownGroup
This parameter is optional.
Allows you to connect certain cooldowns. For example, if one heist with same group has started cooldown, it's not gonna allow you to interact with other heists with same group until heist resets.
CooldownGroup = 'fleeca'
MiddlePoints
These points representing the zone of heist, it's used for loading and unloading data. Render distance for these points are 270.0 units.
MiddlePoints = {
vector3(-1211.15, -335.42, 37.7),
}
Alert
This parameter is optional.
Depends on your dispatch script. (check description below)
Parameters
title:
string
(core, rcore, qs, cd, ps)msg:
string
(origen, qs, cd, ps)code:
string
(core, rcore, ps)flash:
boolean
(cd, qs)blip:
table
sprite:
number
(core, rcore, qs, cd, ps)scale:
number
(core, rcore, qs, cd, ps)colour:
number
(rcore, qs, cd, ps)flashes:
boolean
(rcore, cd, ps)text:
number
(rcore, qs, ps)time:
number
(qs, cd)sound:
number
(cd)
Example
Alert = {
title = "Bank Heist In Progress", -- Title of the alert
msg = "There is a robbery happening at the Pacific Bank. Respond immediately!", -- Message of the alert
code = "10-90", -- Code for the alert
flash = true -- Should the alert flash?
blip = {
sprite = 161, -- Icon used on the map
scale = 1.2, -- Size of the blip
colour = 3, -- Colour of the blip
flashes = true, -- Should the blip flash?
text = "Pacific Bank Robbery", -- Text displayed on blip
time = 5, -- Duration for the blip to stay on the map
sound = 1 -- Sound played when the alert is triggered
}
}
Briefing
This parameter is optional.
Allows you to create briefing spots or add briefing into menu.
If you don't use these parameters: coords, distance, label, zone. It will add it to the briefing menu, otherwise it makes it as briefing spot.
Parameters
coords?:
vector3
distance?:
float
Works with coords parameter.
label?:
string
Works with coords parameter.
zone?:
table
(target settings)length?:
float
width?:
float
minZ?:
float
maxZ?:
float
text:
table
title:
string
description:
string
task:
string
items:
string
image:
string
Example
Briefing = {
coords = vec3(0.0, 0.0, 25.0),
distance = 1.0,
label = 'Open Briefing',
zone = {
length = 1.0,
width = 1.0,
minZ = 23.0,
maxZ = 25.0
},
text = {
title = "**Yacht**",
description = [[
The yacht is a full-sized yacht/cruiser fitted with a range of luxury features, including one or two helipads.
The vessels feature a large bridge and 3 individual decks, while medium and high-end yachts also include a jacuzzi and a luxury helicopter.
]],
task = "Secure the yacht and retrieve the valuables.",
items = "Keys, Weapons, Safe Codes",
image = '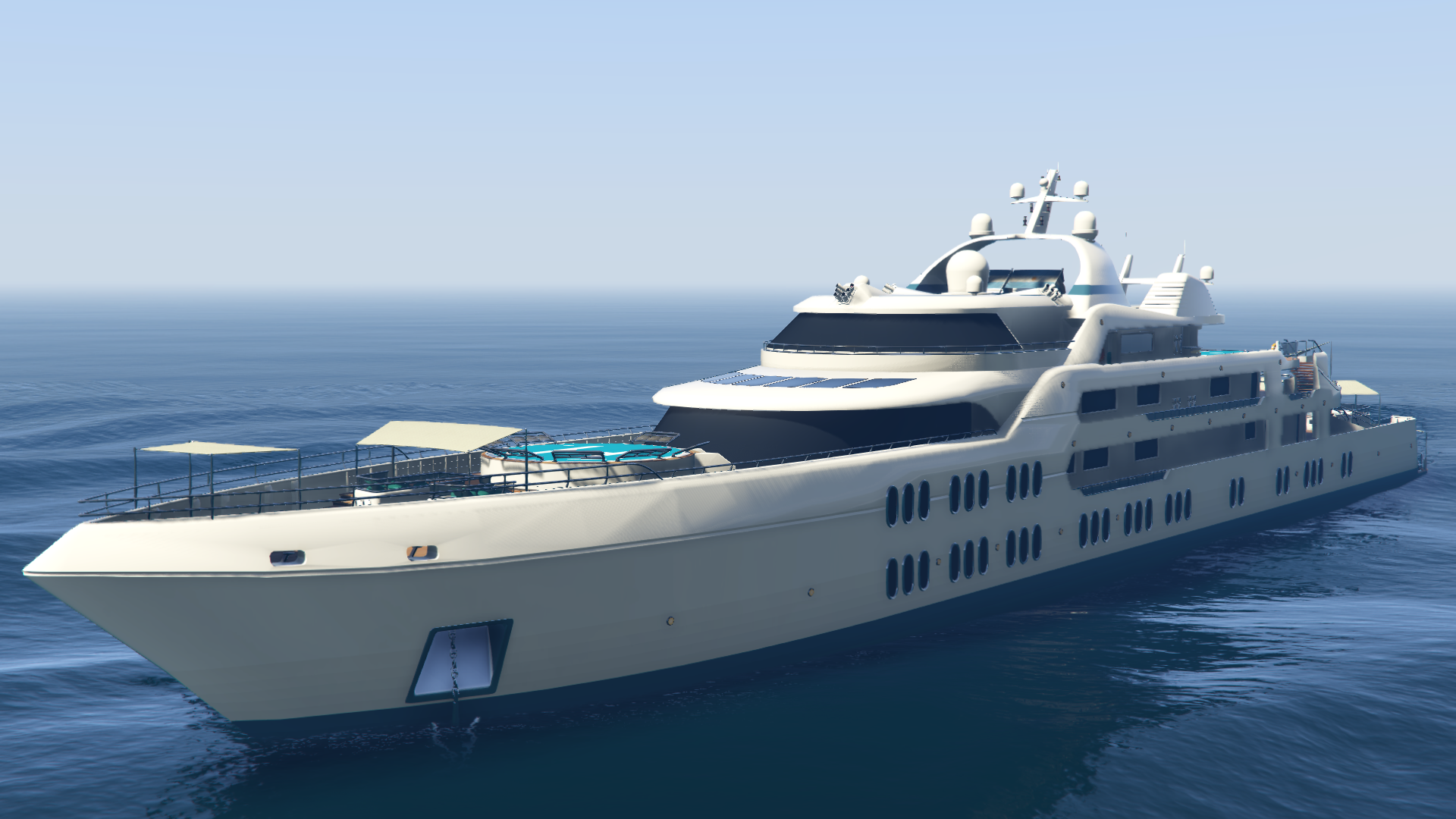'
}
}
Blips
This parameter is optional.
Helpful for marking stuff on the map.
Example
Blips = {
[1] = { -- Index of blip
label = "Name of Blip",
coords = vec3(0.0, 0.0, 0.0),
sprite = 1,
colour = 1,
alpha = 250.0,
scale = 1.0
}
}
Doorlocks
This parameter is optional.
Only for qb and ox doorlock.
-- Numbers or strings representing door locks
Doorlocks = { 1, 'doorlock_name' }
DoorSystem
This parameter is optional.
Built-in door system for objects.
Parameters
coords:
vector3
heading:
table
open:
float
closed:
float
open:
boolean
speed?:
float
(default 0.5)
Example
DoorSystem = {
[1] = { -- Index of door, always start from 1
coords = vec3(0.0, 0.0, 0.0), -- X Y Z, Coordinates of the door object
heading = {
open = 90.0, -- Heading when door is open
closed = 0.0 -- Heading when door is closed
},
door = `v_ilev_bk_vaultdoor`, -- Object hash of the door
open = false, -- Default state of the door
speed = 0.5 -- Speed at which the door opens/closes
}
}
Triggers
This parameter is optional.
Allows you to create reactive zones or spots. They can be one-time or infinite times.
You can make it as polyzone or position.
Parameters
polyzone?:
table
vectors:
table
thickness:
float
debug:
boolean
position?:
table
coords:
vector3
radius:
float
onEnter or onExit:
table
cooldown:
boolean
Check for cooldown.
cops:
boolean
Check for active cops.
trigger?:
boolean
If it's set to true, it will set the trigger to one-time.
Dialog?:
table
title:
string
msg:
string
confirm?:
string
decline?:
string
Functions:
table
Example
Triggers = {
[1] = { -- Index of trigger, always start from 1
polyzone = {
vectors = {
vec3(0.0, 0.0, 0.0), vec3(0.0, 0.0, 0.0),
vec3(0.0, 0.0, 0.0), vec3(0.0, 0.0, 0.0)
},
thickness = 1.0, -- Thickness of the polyzone
debug = true -- Debug mode for polyzone
},
onEnter = {
cooldown = true, -- Cooldown period for the trigger
cops = true, -- Number of cops required for the trigger
Dialog = {
title = "Enter the Zone", -- Title of the dialog
msg = "You are entering a restricted area. Proceed with caution.", -- Message of the dialog
confirm = "Confirm", -- Confirmation text
decline = "Decline" -- Decline text
},
Functions = {
[1] = { -- Index
type = "event", -- function or event
eventType = "client", -- Event type (server or client)
name = "sh-heists:EventName", -- Name of the function or event
params = {} -- Parameters for the function or event
},
[2] = { -- Index
type = "function", -- function or event
name = "START", -- Name of the function or event
params = {} -- Parameters for the function or event
},
}
}
}
}
Checkpoints & Actions
This parameter is optional.
Parameters
coords:
vector4
Designated location.
distance?:
float
Minimum distance for interacting.
label?:
string
Displayed text in target or 3dtext
spawn:
string or number
(only and required for action spots)Callsign for enabling/spawning action spot.
Can be triggered through function list. (Functions)
If you set it to 'MAIN' it will enable/spawn the action spot instantly.
state?:
number
(only for action spots)You can set default state for action spot.
0 = completed, 1 = incompleted
Usable for action spots that you want to call/spawn, but unlock later.
disable_zone?:
boolean
(only for action spots)Disables target zone completely.
Usable when you creating action spot with vehicle.
zone?:
table
(only for target users)length:
float
width:
float
minZ:
float
maxZ:
float
require?:
table
Parameters for checking items, weapons, bag, certain time or you can create your own function.
item?:
string
name:
string
(or table, depends on your inventory)remove?:
boolean
armed?:
table
weapon?:
hash
type?:
string
bag?:
boolean
time?:
table
min_hour:
number
max_hour:
number
custom?:
function
Always return true or false.
rewards?:
table
(only for action spots)item:
string
chance:
number
amount:
table
from:
number
to:
number
reward_limit?:
number
(only for action spots)progressbar?:
table
label:
string
time:
number
In seconds.
canCancel?:
boolean
(default true)anim?:
table
animDict:
string
name:
string
flags?:
number
scenario?:
table
name:
string
playEnter?:
boolean
breakdoor?:
number or string
Works only with ox and qb doorlock.
minigame?:
table
type:
string
difficulty?:
number
Works with 'lockpick' and 'mini_drill'
Only from 1 to 3
speed?:
number
Works with 'datacrack'
Any number should work, default 50-70
time?:
number
Works with:
'blocks' in seconds!
'fingerprint' in seconds!
'fingerprint2' in minutes!
lives?:
number
Works with 'fingerprint'
Always start from 1 to any
scene?:
table
type:
string
loot_obj?:
boolean
offset?:
vector3
coords?:
vector3
rot?:
vector3
object?:
table
(only for action spots)name:
hash
delete?:
boolean
Should it delete after finishing action?
Default is true.
vehicle?:
table
(only for action spots)model:
hash
locked?:
boolean
freeze?:
boolean
onStart? or onSuccess? or onFail?:
table
Functions?:
table
index:
table
type:
string
'function' or 'event'
name:
string
Name of function or event name.
eventType?:
string
Only if type is 'event'
'client' or 'server'
params:
table
delay:
number
Example of checkpoint
Checkpoints = {
[1] = { -- Index of checkpoint
coords = vec4(0.0, 0.0, 0.0, 0.0), -- Vector4 coordinates
zone = { -- Target settings (only for qb and ox target)
minZ = 1.0, -- Minimum Z coordinate
maxZ = 2.0, -- Maximum Z coordinate
length = 3.0, -- Length of the zone
width = 4.0 -- Width of the zone
},
label = "Checkpoint Label", -- Text label for the checkpoint
distance = 5.0, -- Distance for the checkpoint
breakdoor = "doorlock_id_or_name", -- ID or name of doorlock
require = {
item = {
name = "required_item", -- Name of required item
remove = true -- Should the item be removed after finishing action?
},
bag = true -- Is a bag required?
},
minigame = {
type = "minigame_name" -- Name of the minigame
},
scene = {
type = "scene_name", -- Name of the scene
offset = vec3(0.0, 0.0, 0.0), -- Vector3 offset coordinates
coords = vec3(0.0, 0.0, 0.0), -- Vector3 coordinates
rot = vec3(0.0, 0.0, 0.0) -- Vector3 rotation
}
}
}
Example of action spot
Actions = {
[1] = { -- Index of action spot
coords = vec4(0.0, 0.0, 0.0, 0.0), -- Vector4 coordinates
zone = { -- only for qb and ox target
minZ = 1.0, -- Minimum Z coordinate
maxZ = 2.0, -- Maximum Z coordinate
length = 3.0, -- Length of the zone
width = 4.0 -- Width of the zone
},
spawn = "MAIN", -- ID or name for enabling action spot
label = "Label", -- Text label
distance = 1.0, -- Distance
breakdoor = "name_of_doorlock", -- ID or name of doorlock
require = {
item = {
name = "required_item", -- Name of required item
remove = true -- Should the item be removed after finishing action?
},
bag = true -- Is a bag required?
},
minigame = {
type = "fingerprint" -- Name of the minigame
},
scene = {
type = "name", -- Name of the scene
offset = vec3(0.0, 0.0, 0.0), -- Vector3 offset coordinates
},
object = {
name = `v_ilev_bk_vaultdoor`, -- Hash name of the object
delete = true -- Should the object be deleted?
},
rewards = {
[1] = {
item = "reward_item", -- Name of the item
chance = 50, -- Chance to receive the reward
amount = {
from = 1, -- Minimum amount
to = 5 -- Maximum amount
}
}
},
onSuccess = {
Functions = {
[1] = {
type = "event", -- Type of function (function or event)
eventType = "server", -- Event type (server or client)
name = "sh-heists:NameOfEvent", -- Name of the function
params = {} -- Parameters
}
}
}
}
}
NPC
This parameter is optional.
NPC = {
FriendlyGuards = boolean,
GLOBAL_SETTINGS = table,
NPCs = table
}
NPC Parameters
coords:
vector4
Doesn't work with global settings.
Spawning location of npc.
spawn:
string or number
Callsign/name for spawning NPC, can be triggered by function list.
If you set it to 'MAIN' it will spawn the npc instantly.
skin:
hash
state?:
number
weapon?:
hash
freeze?:
boolean
Freezes entity at position
movement?:
table
Types:
0 - Stationary (Will jsut stand in place)
1 - Defensive (Will try to find cover and very likely to blind fire)
2 - Offensive (Will attempt to charge at enemy but take cover as well)
3 - Suicidal (Will try to flank enemy in suicidal attack)
from:
number
to:
number
combat_ability?:
table
Types:
0 - Poor
1 - Average
2 - Professional
from:
number
to:
number
animation?:
table
dict?:
string
anim?:
string
flag?:
number
scenario?:
string
walk_around?:
boolean
Doesn't work with global settings.
Can't be used with patrol parameter.
fire_pattern?:
hash
Weapon fire pattern.
alertness?:
table
Types:
0 - Neutral
1 - Heard something (gun shot, hit, etc)
2 - Knows (the origin of the event)
3 - Fully alerted
from:
number
to:
number
highly_perceptive?:
boolean
Instantly knows about enemy.
hearing_range?:
float
peripheral_range?:
float
combat_range?:
number
Defines the scope within which the npc will engage in combat with the target.
Types:
0 - Near (5-15m)
1 - Medium (7-30m)
2 - Far (15-40m)
3 - Very Far (22-45m)
accuracy?:
number
Starts from 0 to 100 (being perfectly accurate)
always_fight?:
boolean
block_events?:
boolean
Doesn't react to any events like shooting etc.
health?:
number
armor?:
number
bloody_clothes?:
boolean
walkstyle?:
string
patrol?:
table
name:
string
Must be unique name.
anim:
'StandGuard' or 'WORLD_HUMAN_GUARD_STAND'
wait:
number
In miliseconds.
positions:
table
Vector3
disable_speaking?:
boolean
disable_ragdoll?:
boolean
disable_headshot?:
boolean
disable_weap_switch?:
boolean
fire_resistance?:
boolean
find_target?:
boolean
Finds target automatically after spawning.
drop_weapon?:
boolean
blip?:
boolean
Marks npc on map.
onEliminate?:
table
delete?:
boolean
rewards?:
table
item_name:
string
from:
number
to:
number
action?:
table
Functions?:
table
Example
NPC = {
FriendlyGuards = true,
GLOBAL_SETTINGS = { -- if npcs doesn't have certain settings, it will set global settings to him
spawn = 'START',
health = 200,
armor = 100,
state = 5, -- -- 0 = Companion 1 = Respect 2 = Like 3 = Neutral 4 = Dislike 5 = Hate or false
skin = `s_m_m_marine_01`,
weapon = `WEAPON_CARBINERIFLE`,
animation = nil,
walkstyle = false, -- 'MOVE_M@DRUNK@VERYDRUNK',
freeze = false,
block_events = false, -- they will not react to any events like shooting etc
bloody_clothes = false,
fire_pattern = `FIRING_PATTERN_FULL_AUTO`,
fire_resistance = false,
walk_around = false, -- patrol, guard, walk around
highly_perceptive = false,
alertness = {from = 3, to = 3}, -- from 1 to 3
movement = {from = 1, to = 3}, -- randomised - 1 defensive, 2 agressive, 3 suicidal (flank)
combat_ability = {from = 2, to = 2}, -- 0 poor, 1 average, 2 profesional
combat_range = {from = 2, to = 2}, -- 0 near, 1 medium, 2 far
accuracy = 40, -- from 0 to 100
always_fight = true, -- true / false
hearing_range = 150.0, -- units
seeing_range = 150.0, -- units
peripheral_range = 150.0, -- units
disable_speaking = false,
disable_ragdoll = false,
disable_headshot = false,
disable_weap_switch = false,
find_target = true,
},
NPCs = { -- npcs to spawn
[1] = { coords = vector4(514.02, 4878.47, -62.59, 261.2) }
},
}
Functions
This parameter is optional.
Main?:
function
Start?:
function
End?:
function
Full Config Example
CFG.Heists["Pacific"] = {
RequiredCops = 0, -- number of cops required to start
Cooldown = 120, -- min -- global bank cooldown
Alert = {
title = 'Bank Robbery in Progress', -- core, rcore, qs, cd, ps
msg = 'A %s robbing at %s', -- origen, qs, cd, ps
code = '10-XX', -- core, rcore, ps
flash = 0, -- cd, qs
blip = {
sprite = 431, -- core, rcore, qs, cd, ps
scale = 1.2, -- core, rcore, qs, cd, ps
colour = 49, -- rcore, qs, cd, ps
flashes = true, -- rcore, cd, ps
text = '911 - Robbery in Progress', -- rcore, qs, ps
time = (5*60*1000), -- qs, cd
sound = 1 -- cd
}
},
-- it will lock these doors when heist resets
Doorlocks = {1, 2, 3, 6},
-- built-in door system
DoorSystem = {
[1] = {
coords = vector3(254.15, 225.12, 101.88),
heading = {open = 10.0, closed = 159.96},
speed = 0.5,
door = `v_ilev_bk_vaultdoor`,
open = false
},
},
Briefing = {
text = {
title = '**Pacific Standard Bank**',
description = [[
The Pacific Standard Public Deposit Bank is a public deposit bank,
located in Downtown Vinewood, Los Santos.
The bank has two entrances, one at the intersection of Vinewood Boulevard and Alta Street,
and a second to the east exiting onto Vinewood Boulevard.
Just inside both entrances are banks of usable Automated Teller Machines.
]],
task = 'Break in, drill safe, steal everything you can and escape.',
items = '1x thermite, 2x torch, 3x drill, 1x electronickit',
image = '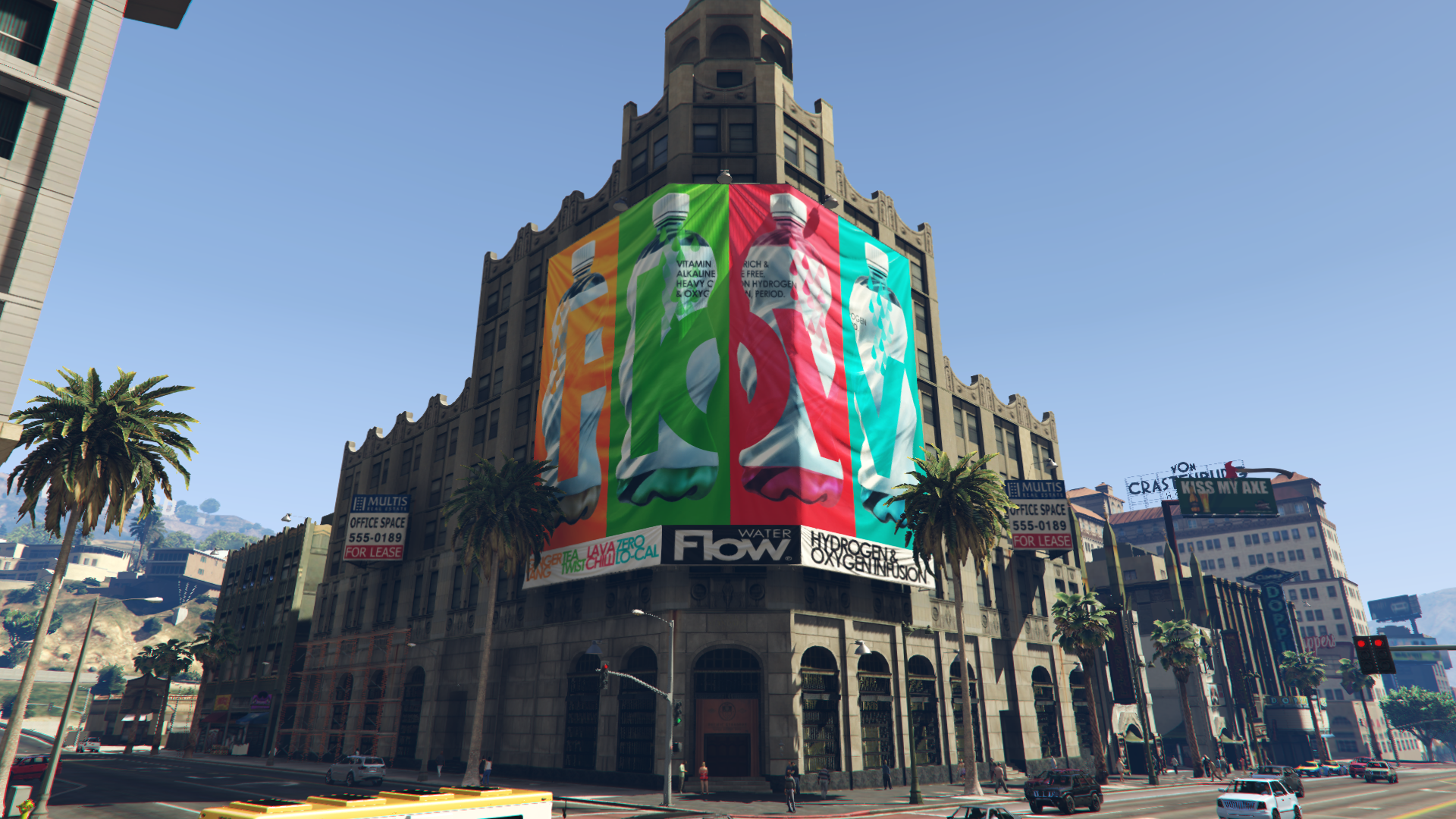' -- name and url
},
},
Checkpoints = {
[1] = {
coords = vector4(257.04, 219.85, 106.29, 342.86), -- x y z heading
zone = {minZ = 105.0, maxZ = 107.3, length = 1.0, width = 0.9},
label = 'Break Door',
require = {
item = { name = 'thermite', remove = true },
bag = false
},
scene = {type = 'thermite2', offset = vec3(0.32, 0.40, 0.0)},
breakdoor = 6,
onStart = {
Functions = {
[1] = { type = 'function', name = 'SET_NPC_STATE', params = { state = 5 } },
}
},
onSuccess = {
Functions = {
[1] = { type = 'function', name = 'START' },
[2] = { type = 'function', name = 'CALL_ALERT' },
[3] = {
type = 'function', name = 'UPDATE_MODEL',
params = {
coords = vec3(256.31, 220.66, 106.43), radius = 0.5,
model = `hei_v_ilev_bk_gate_pris`, newModel = `hei_v_ilev_bk_gate_molten`
}
},
[4] = {
type = 'function', name = 'ALARM',
params = {
coords = vector3(256.79, 219.87, 106.29),
duration = 300 -- seconds
}
},
}
}
},
[2] = {
coords = vector4(261.92, 223.11, 106.28, 251.0), -- x y z heading
zone = {minZ = 105.0, maxZ = 107.3, length = 1.2, width = 1.2},
distance = 1.5,
label = 'Break Door',
require = {
item = { name = 'electronickit', remove = true },
bag = false
},
scene = { type = 'laptop', offset = vec3(0.0, 0.35, -0.05) },
minigame = {type = 'datacrack'},
breakdoor = 1,
onSuccess = {
Functions = {
[1] = {
type = 'function', name = 'LASER',
params = {
matrix = {
vec3(-0.934, 0.356, -0.0), vec3(0.356, 0.934, 0.0),
vec3(0.0, 0.0, 1.0), vec3(261.221, 224.835, 101.6),
},
settings = {
uniqueId = 696969, length = 5, distance = 10.0,
onTouch = {
LockActions = {1}, -- action ids
Functions = {
[1] = { type = 'function', name = 'CALL_ALERT' },
[2] = {
type = 'function',
name = 'START_GAS',
params = {
unique_id = 2,
timer = 0, -- minutes
locations = {
vector3(263.54, 212.2, 101.68),
vector3(256.05, 215.38, 101.68),
vector3(248.75, 218.16, 101.68),
vector3(257.2, 225.95, 101.88)
}
}
},
}
}
}
}
},
}
}
},
[3] = {
coords = vector4(254.17, 224.92, 101.88, 156.35), -- x y z heading
zone = {minZ = 100.5, maxZ = 103.0, length = 1.2, width = 1.7, heading = 160.0},
label = 'Use Drill',
require = {
item = { name = 'drill', remove = true },
bag = false,
-- time = {min_hour = 7, max_hour = 20 },
},
scene = { type = 'drill', offset = vec3(0.0, 0.35, 0.0) },
minigame = {type = 'big_drill'},
onSuccess = {
Functions = {
[1] = {
type = 'function',
name = 'DOOR',
params = {
id = 1,
open = true
}
},
}
}
},
},
Actions = {
[1] = {
coords = vector4(264.89, 219.89, 101.68, 304.17), -- x y z heading
zone = {minZ = 100.5, maxZ = 102.5, length = 1.7, width = 1.3, heading = 304.0},
label = 'Hack Security',
spawn = 'START',
minigame = { type = 'password' },
scene = { type = 'type', offset = vec3(0.0, 0.8, 0.15) },
onSuccess = {
Functions = {
[1] = {
type = 'function',
name = 'REMOVE_LASER',
params = {
id = 696969
}
},
}
}
},
[2] = {
coords = vector4(252.32, 221.26, 101.68, 163), -- x y z heading
zone = {minZ = 100.5, maxZ = 103.0, length = 0.7, width = 1.8, heading = 160.0},
label = 'Use Torch',
spawn = 'START',
scene = { type = 'torch', offset = vec3(1.02, 2.1, -0.05) },
breakdoor = 2
},
[3] = {
coords = vector4(261.25, 215.34, 101.68, 257), -- x y z heading
zone = {minZ = 100.5, maxZ = 103.0, length = 0.7, width = 1.5, heading = 249.72},
label = 'Use Torch',
spawn = 'START',
scene = { type = 'torch', offset = vec3(1.3, 2.05, -0.05) },
breakdoor = 3
},
-- LOOT
[4] = {
coords = vector4(266.09, 213.54, 101.68, 260.76), -- x y z heading
zone = {minZ = 100.5, maxZ = 103.0, length = 0.7, width = 1.5, heading = 249.72},
label = 'Use Drill',
spawn = 'SPART',
require = {
item = { name = 'drill', remove = true },
bag = false
},
rewards = {
{ item = 'gold_ring', chance = 100, amount = { from = 10, to = 15 } },
{ item = 'diamond_ring', chance = 100, amount = { from = 10, to = 15 } },
{ item = 'goldchain', chance = 100, amount = { from = 10, to = 15 } },
{ item = 'diamond_necklace', chance = 100, amount = { from = 10, to = 15 } },
},
scene = { type = 'drill', offset = vec3(0.03, 0.29, 0.0) },
minigame = {type = 'mini_drill'},
},
[5] = {
coords = vector4(257.62, 215.02, 100.68, 358.55), -- x y z heading
zone = {minZ = 100.5, maxZ = 102.0, length = 0.7, width = 1.1},
label = 'Grab Gold',
spawn = 'START',
require = {
bag = false
},
rewards = {
{ item = 'goldbar', chance = 100, amount = { from = 1, to = 1 }, trolley = true },
},
scene = { type = 'gold_trolley2', offset = vec3(0.0, 0.0, 0.0) },
object = { name = `ch_prop_gold_trolly_01a`, delete = false }
},
[6] = {
coords = vector4(259.92, 217.27, 100.68, 151.47), -- x y z heading
zone = {minZ = 100.5, maxZ = 102.0, length = 0.7, width = 1.1},
label = 'Grab Money',
spawn = 'START',
require = {
bag = false
},
rewards = {
{ item = 'markedbills', chance = 100, amount = { from = 1000, to = 1000 }, trolley = true },
},
scene = { type = 'money_trolley2', offset = vec3(0.0, 0.0, 0.0) },
object = { name = `hei_prop_hei_cash_trolly_01`, delete = false }
},
},
NPC = {
FriendlyGuards = true, -- only with pre-defined jobs in main config file
GLOBAL_SETTINGS = { -- if npcs doesn't have certain settings, it will set global settings to him
spawn = 'MAIN',
health = 200,
armor = 0,
state = 4, -- -- 0 = Companion 1 = Respect 2 = Like 3 = Neutral 4 = Dislike 5 = Hate or false
skin = `s_m_m_security_01`,
weapon = `WEAPON_COMBATPISTOL`,
animation = {scenario = 'WORLD_HUMAN_GUARD_STAND'},
walkstyle = false, -- 'MOVE_M@DRUNK@VERYDRUNK',
freeze = false,
block_events = false, -- they will not react to any events like shooting etc
bloody_clothes = false,
fire_resistance = false,
walk_around = false, -- patrol, guard, walk around
highly_perceptive = false,
alertness = {from = 3, to = 3}, -- from 1 to 3
movement = {from = 1, to = 1}, -- 1 defensive, 2 agressive, 3 suicidal (flank)
combat_ability = {from = 0, to = 2}, -- 0 poor, 1 average, 2 profesional
combat_range = {from = 0, to = 2}, -- 0 near, 1 medium, 2 far
accuracy = 35, -- from 0 to 100
always_fight = true, -- true / false
hearing_range = 40.0, -- units
seeing_range = 150.0, -- units
peripheral_range = 150.0, -- units
disable_speaking = false,
disable_ragdoll = false,
disable_headshot = false,
disable_weap_switch = false,
find_target = false,
},
NPCs = {
-- guards --
[1] = { coords = vector4(241.3, 216.69, 106.29, 300.76) },
[2] = { coords = vector4(238.93, 221.26, 106.29, 259.3) },
[3] = { coords = vector4(259.68, 218.1, 106.29, 102.06) },
[4] = { coords = vector4(238.07, 218.14, 110.28, 299.28) },
[5] = { coords = vector4(252.58, 217.55, 101.68, 350.88), spawn = 'START', state = 5, find_target = true },
[6] = { coords = vector4(263.43, 221.47, 101.68, 302.71), spawn = 'START', state = 5, find_target = true },
-- civs --
},
},
Functions = {
End = function(heist) -- end of heist, for all
TriggerEvent(EVENT_PREFIX..'CL:RESTORE_MODEL',
vec3(256.31, 220.66, 106.43), 0.5,
`hei_v_ilev_bk_gate_pris`, `hei_v_ilev_bk_gate_molten`
)
end,
},
}
Last updated